The Subquery in a SELECT Statement
- A subquery is a SELECT statement coded within another SELECT statement.
- A subquery can return a single value or a list of values.
- A subquery can return multiple columns.
- A subquery cannot make use of the ORDER BY clause
- A subquery can be nested within another subquery
- You can use a subquery in a WHERE, HAVING, FROM and SELECT clause.
Code Sample:
1 USE world;
2 SELECT name, population
3 FROM city
4 WHERE CountryCode IN
5 (SELECT code
6 FROM country
7 WHERE region = 'Caribbean')
8 ORDER BY population
9 LIMIT 5;
Results:
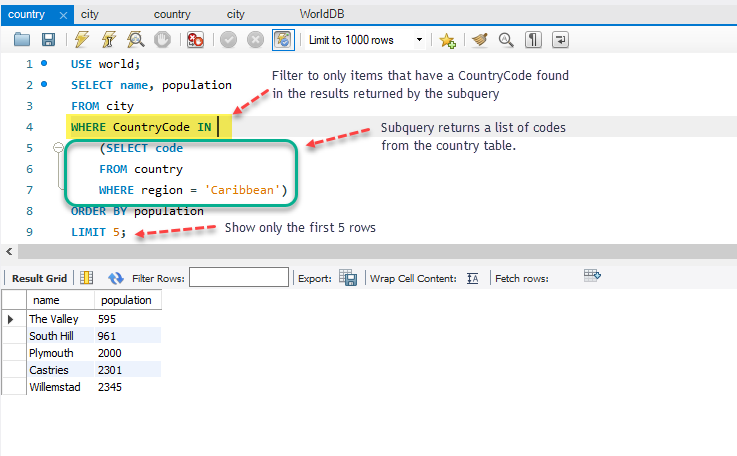
SELECT name, population
FROM city
- Return the name and population columns from the city table.
WHERE CountryCode IN
- Filter to only rows that have a value found in the subsequent subquery.
(SELECT code
FROM country
WHERE region = 'Caribbean')
- The subquery shown above returns a result list of all of the codes from the country table that have a region of ‘Caribbean’.
- The subquery must be in parentheses in MySQL.
- Each code (PK in country table) returned by the subquery is checked against CountryCode (FK in city table). If they match, the name and population are retrieved from the city table.